Difficulty: Beginner
Bluetooth modules such as the HC-05 allows makers an easy to integrate solution for adding Bluetooth capability to projects. These modules can be configured using AT commands and a home made programmer. In this tutorial I will show you how to make a Bluetooth programmer that can be used with HC-05 Bluetooth modules.
The below diagram shows the interconnections for the BT programmer;
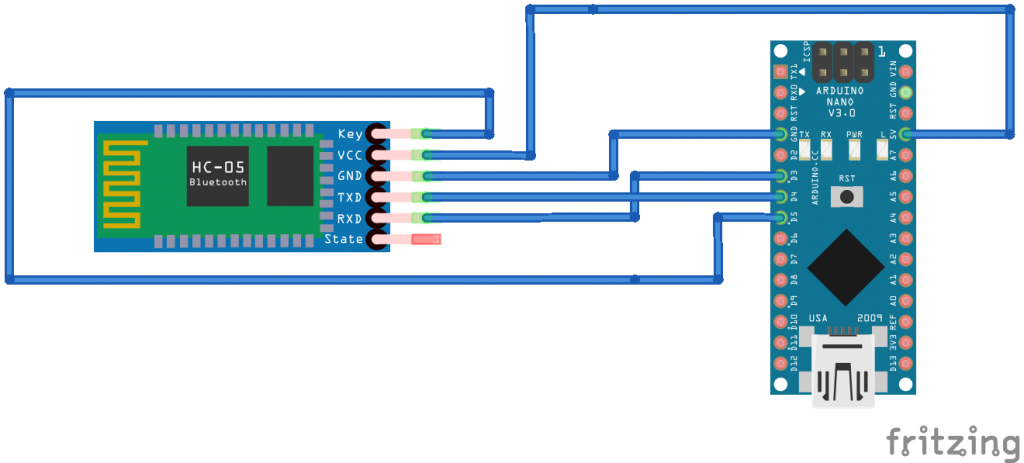
If you don’t want to make the above circuit you can go to our E-Bay shop and buy a BT Programmer PCB with headers attached, you will just need to add an Arduino Nano V3 and upload the below code to the micro-controller. The below picture shows the PCB layout;
In order to program a BT module you need to give it 5v, GND and attach the TX and RX pins to digital IO pins on the Arduino. In this example I decided to use pins 4 and 3. I also used pin 5 to put a logic high on the EN pin of the HC-05. This will put the HC-05 into AT command mode as soon as its inserted into the programmer so you don’t need to mess about with the EN button. For this to work the programmer should be powered up then the HC-05 inserted after.
Once you’ve made the above circuit upload the below code to the Arduino;
The above code creates a software serial connection to the HC-05. this will allow you to pass AT commands from the Arduino’s serial port to the HC-05. Please note that the baud rate of the HC-05 is 38400 if you use a different Bluetooth module this may differ. This baud rate is the AT command mode baud rate and can not be altered; it is different to the UART baud rate that can be altered for Bluetooth communication. In order to program the HC-05 open the serial monitor, set the baud rate to 57600 (this is the baud of the hardware serial port) and type “AT”, if the serial monitor returns OK then the HC-05 is ready for programming. I advise that you insert the HC-05 after the programmer has been powered up this will allow the HC-05 to go into AT command mode straight away.
The main loop of the program listens for commands from the serial monitor and relays them to the software serial port and vice versa allowing you to have full duplex communications with the HC-05 enabling it to be programmed via AT commands.
This document HC-05 AT Commands contains a list commands that can be used with the HC-05.
The below video shows how to upload code to the programmer and how to use AT commands;